How to set selected images for UITabBarItem in UITabBarController
There is a bug/feature in the UITabBarController
class. Set up selected images for tab bar items using
the Interface Builder:
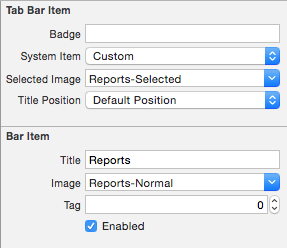
Run your application and look into the Console in the Debug area. You should see the following error message:
2014-12-14 20:50:52.889 UITabBarControllerBugOrFeature[12419:600151] CUICatalog: Invalid asset name supplied: (null) 2014-12-14 20:50:52.890 UITabBarControllerBugOrFeature[12419:600151] CUICatalog: Invalid asset name supplied: (null) 2014-12-14 20:50:52.890 UITabBarControllerBugOrFeature[12419:600151] Could not load the "(null)" image referenced from a nib in the bundle with identifier "com.manenko.UITabBarControllerBugOrFeature"
It seems, that application can’t find image resource because of some unknown reason. But it is there!.. Doh :[
The fix is simple: you should assign selected images to corresponding UITabBarItem
in code. And don’t forget to clear
Selected Image field in the Attributes Inspector.
The best place to assign images is AppDelegate
class:
func application(application: UIApplication, didFinishLaunchingWithOptions launchOptions: [NSObject: AnyObject]?) -> Bool {
let controller = window?.rootViewController as UITabBarController
(controller.tabBar.items![0] as UITabBarItem).selectedImage = UIImage(named: "Image1-Selected")
(controller.tabBar.items![1] as UITabBarItem).selectedImage = UIImage(named: "Image2-Selected")
(controller.tabBar.items![2] as UITabBarItem).selectedImage = UIImage(named: "Image3-Selected")
return true
}
And if you want to change tint color of the tab bar, you also should do this in code. Just add the following line to the
previous method before the return
statement:
controller.tabBar.tintColor = UIColor.orangeColor()
That’s it. I hope this was useful.